Rain in Bergen#
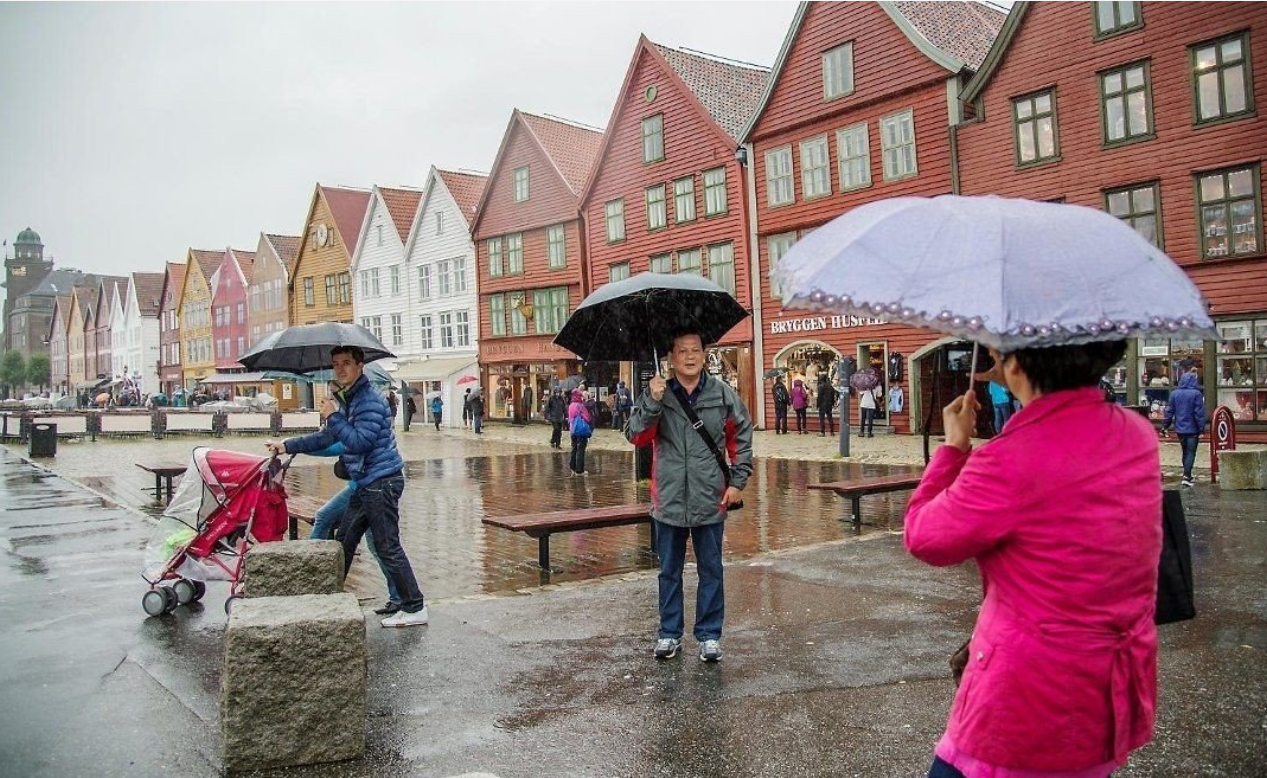
Bergen is famous for having a lot of rain. So much so that the Bergen bunad features umbrella-themed silver:
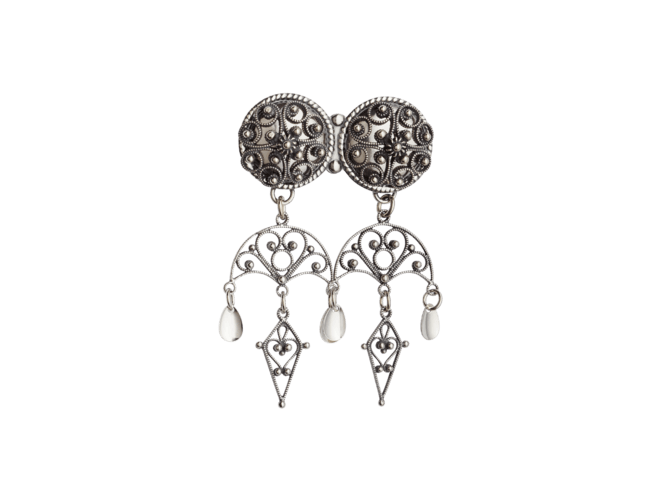
To practise get
requests, json
and plotting, we are going to make a bar plot of the rain in Bergen by month. To do so, we will access historical data from MET.
Frost API#
The Frost API from MET provides historical weather and climate data of e.g. temperature, precipitation and wind.
Step 1
Check the conditions for using this API.
What rules do they have for using the API?
Step 2
Complete the following code snippet so that it gets the precipitation per day in Bergen
You can use the following parameters for the get request
Key |
Value |
---|---|
‘sources’ |
‘SN50540’ |
‘elements’ |
‘sum(precipitation_amount P1D)’ |
‘referencetime’ |
‘2020-01-01/2020-12-31’ |
Blindern has the code SN18700
import requests
import json
# Insert your own client ID here
client_id = ...
# Define url for the get request
url = 'https://frost.met.no/observations/v0.jsonld'
# Define parameters for the get request (as a dictionary)
parameters = { ... }
# Issue an HTTP GET request
r = requests.get(url, parameters, auth=(client_id,''))
# Check if the request worked, print out any errors
if r.status_code ...:
...
Step 3:
Parse through the data. Make a dictionary of the total rain by month.
prec_per_month = {}
data = r.json() ...
for i in range(len(data)):
month = ...
prec = ...
if month in prec_per_month:
prec_per_month[month] += prec
else:
prec_per_month[month] = prec
tot_prec = prec_per_month.values()
months = prec_per_month.keys()
Next we want to plot this data in a bar plot.
To do this we use pyplot:
import matplotlib.pyplot as plt
fig = plt.figure()
ax = plt.axes()
In pyplot, the figure (an instance of the class plt.Figure
) can be thought of as a single container that contains all the objects representing axes, graphics, text and labels. The axes (an instance of the class plt.axes
) is more or less what is shown above: a bounding box with ticks and labels, which we will fill with plot elements.
We want to add a bar plot to ax
ax.bar?
Step 4
Make a bar plot showing the total precipitation each month.
ax.bar(...)
Step 5
Format the bar plot so that the xticks show the abbreviated month name.
Tip: Use the calendar package.
import calendar
import time
xticks = ... # this should be a list of xtick values
xtick_vals = ... # this should be a list of the corresponding xtick labels
ax.set_xticks(xticks)
ax.set_xticklabels(xtick_vals)
fig